Here's an example of a Table UI Design created using pure CSS.
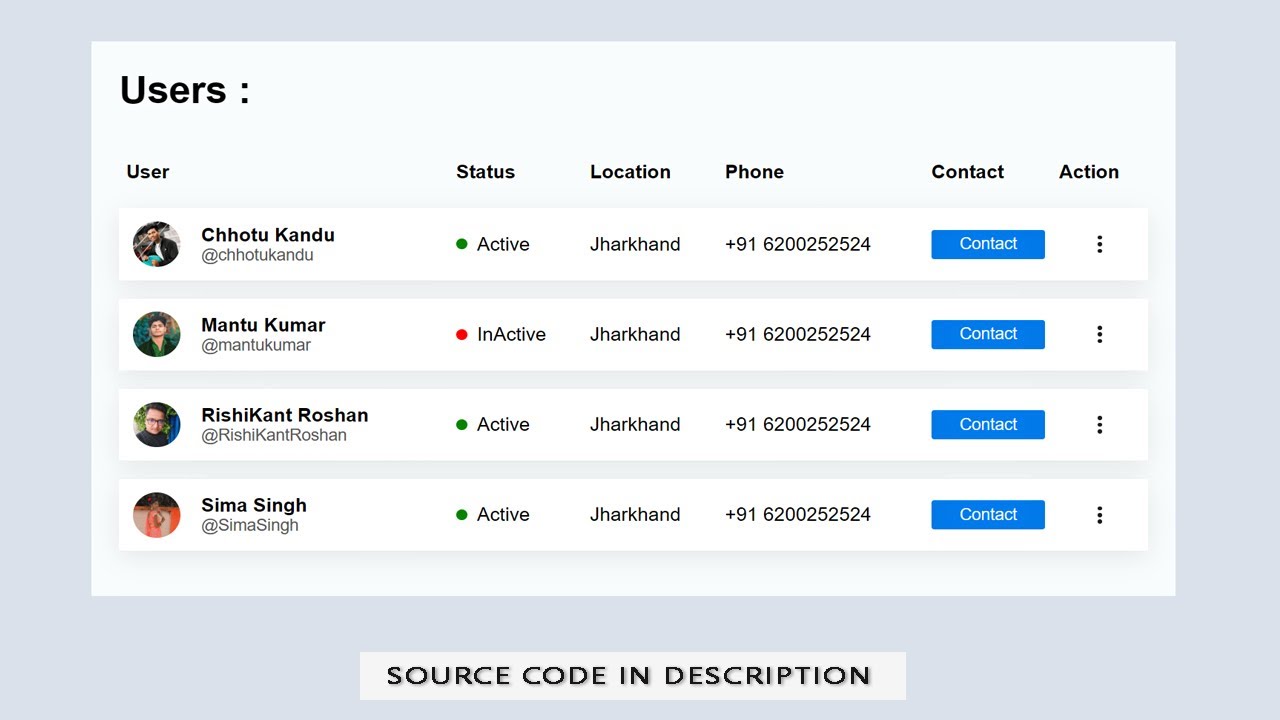
1. Create an HTML file and include a basic structure with a head and body tag. You can use the following code as a starting point and Add the CSS files to your HTML document.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Create Table UI Design in CSS</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
</body>
</html>
2. Inside the body tag, create a div element with a class of "container".
<div class="container">
<h1 class="title">Users : </h1>
<table class="table">
<thead class="thead">
<tr class="list">
<th class="user">User</th>
<th class="status">Status</th>
<th class="location">Location</th>
<th class="phone">Phone</th>
<th class="contact">Contact</th>
<th class="action">Action</th>
</tr>
</thead>
<tbody class="tbody">
<tr class="list">
<th class="user">
<img src="user-image/Chhotu Kandu.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">Chhotu Kandu</div>
<div class="user-name">@chhotukandu</div>
</div>
</th>
<th class="status">
<div class="active">Active</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
<tr class="list">
<th class="user">
<img src="user-image/Mantu Kumar.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">Mantu Kumar</div>
<div class="user-name">@mantukumar</div>
</div>
</th>
<th class="status">
<div class="inactive">InActive</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
<tr class="list">
<th class="user">
<img src="user-image/RishiKant Roshan.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">RishiKant Roshan</div>
<div class="user-name">@RishiKantRoshan</div>
</div>
</th>
<th class="status">
<div class="active">Active</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
<tr class="list">
<th class="user">
<img src="user-image/Sima Singh.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">Sima Singh</div>
<div class="user-name">@SimaSingh</div>
</div>
</th>
<th class="status">
<div class="active">Active</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
</tbody>
</table>
</div>
3. Create a CSS file and style your Table to make it look nice. You can use the following code as a starting point:
* {
box-sizing: border-box;
}
body {
background: #e0e7f1;
font-family: sans-serif;
font-size: 14px;
}
.container {
max-width: 780px;
background: #f9fdfe;
padding: 20px;
margin: 0 auto;
margin-top: 40px;
}
h1 {
margin-top: 0;
}
table {
text-align: left;
width: 100%;
border-collapse: separate;
border-spacing: 0 1em;
}
thead th {
font-weight: 600;
}
th {
padding: 5px;
font-weight: 400;
}
tbody .list {
background: #fff;
box-shadow: 0 5px 20px #0001;
}
tbody .user {
display: flex;
align-items: center;
}
tbody .icon {
width: 34px;
height: 34px;
margin: 5px;
background: #eee;
border-radius: 50%;
margin-right: 15px;
}
tbody .name {
font-weight: 600;
}
tbody .user-name {
font-size: 12px;
color: #666;
}
tbody .active,
tbody .inactive {
padding-left: 15px;
position: relative;
}
tbody .active::before,
tbody .inactive::before {
content: "";
position: absolute;
width: 8px;
height: 8px;
border-radius: 50%;
left: 0;
top: 25%;
background: #079400;
}
tbody .inactive::before {
background: #f00;
}
.button {
padding: 4px 10px;
background: #0084ff;
color: #fff;
border-radius: 2px;
font-size: 12px;
text-align: center;
}
.button:hover {
cursor: pointer;
background: #0060ba;
}
.action-button {
position: relative;
width: 20px;
height: 20px;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
margin: 0 auto;
cursor: pointer;
}
.action-button span {
display: block;
width: 3px;
height: 3px;
background: #222;
border-radius: 50%;
margin: 1px;
}
.action-button:hover span {
background: #0084ff;
}
4. Save the file and open it in a web browser to see your Table UI Design.
<div class="container">
<h1 class="title">Users : </h1>
<table class="table">
<thead class="thead">
<tr class="list">
<th class="user">User</th>
<th class="status">Status</th>
<th class="location">Location</th>
<th class="phone">Phone</th>
<th class="contact">Contact</th>
<th class="action">Action</th>
</tr>
</thead>
<tbody class="tbody">
<tr class="list">
<th class="user">
<img src="user-image/Chhotu Kandu.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">Chhotu Kandu</div>
<div class="user-name">@chhotukandu</div>
</div>
</th>
<th class="status">
<div class="active">Active</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
<tr class="list">
<th class="user">
<img src="user-image/Mantu Kumar.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">Mantu Kumar</div>
<div class="user-name">@mantukumar</div>
</div>
</th>
<th class="status">
<div class="inactive">InActive</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
<tr class="list">
<th class="user">
<img src="user-image/RishiKant Roshan.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">RishiKant Roshan</div>
<div class="user-name">@RishiKantRoshan</div>
</div>
</th>
<th class="status">
<div class="active">Active</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
<tr class="list">
<th class="user">
<img src="user-image/Sima Singh.jpg" alt="" class="icon">
<div class="name-or-userName">
<div class="name">Sima Singh</div>
<div class="user-name">@SimaSingh</div>
</div>
</th>
<th class="status">
<div class="active">Active</div>
</th>
<th class="location">Jharkhand</th>
<th class="phone">+91 6200252524</th>
<th class="contact">
<div class="button">Contact</div>
</th>
<th class="action">
<div class="action-button">
<span></span>
<span></span>
<span></span>
</div>
</th>
</tr>
</tbody>
</table>
</div>
* {
box-sizing: border-box;
}
body {
background: #e0e7f1;
font-family: sans-serif;
font-size: 14px;
}
.container {
max-width: 780px;
background: #f9fdfe;
padding: 20px;
margin: 0 auto;
margin-top: 40px;
}
h1 {
margin-top: 0;
}
table {
text-align: left;
width: 100%;
border-collapse: separate;
border-spacing: 0 1em;
}
thead th {
font-weight: 600;
}
th {
padding: 5px;
font-weight: 400;
}
tbody .list {
background: #fff;
box-shadow: 0 5px 20px #0001;
}
tbody .user {
display: flex;
align-items: center;
}
tbody .icon {
width: 34px;
height: 34px;
margin: 5px;
background: #eee;
border-radius: 50%;
margin-right: 15px;
}
tbody .name {
font-weight: 600;
}
tbody .user-name {
font-size: 12px;
color: #666;
}
tbody .active,
tbody .inactive {
padding-left: 15px;
position: relative;
}
tbody .active::before,
tbody .inactive::before {
content: "";
position: absolute;
width: 8px;
height: 8px;
border-radius: 50%;
left: 0;
top: 25%;
background: #079400;
}
tbody .inactive::before {
background: #f00;
}
.button {
padding: 4px 10px;
background: #0084ff;
color: #fff;
border-radius: 2px;
font-size: 12px;
text-align: center;
}
.button:hover {
cursor: pointer;
background: #0060ba;
}
.action-button {
position: relative;
width: 20px;
height: 20px;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
margin: 0 auto;
cursor: pointer;
}
.action-button span {
display: block;
width: 3px;
height: 3px;
background: #222;
border-radius: 50%;
margin: 1px;
}
.action-button:hover span {
background: #0084ff;
}