Here's an example of a Social Media Icon created using pure CSS.
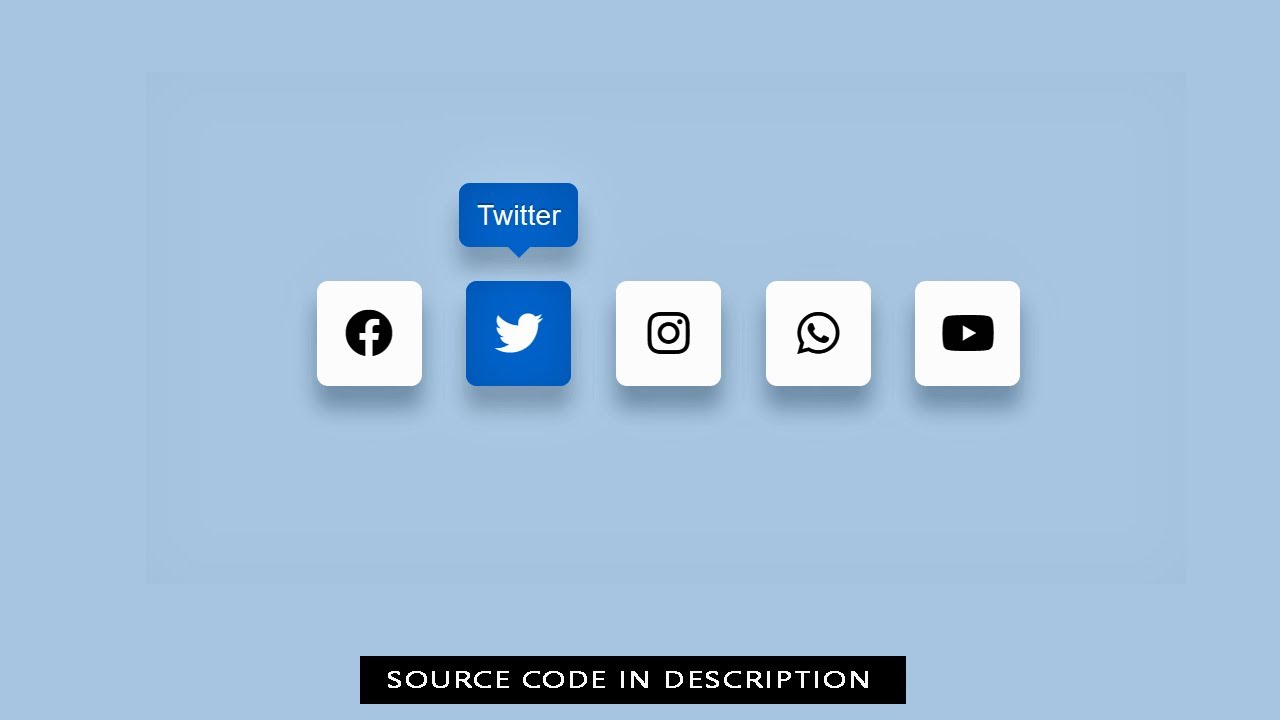
1. Create an HTML file and include a basic structure with a head and body tag. You can use the following code as a starting point and Add the CSS files to your HTML document.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Social Media Icon Ui Design in Css</title>
<link rel="stylesheet" href="style.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css">
</head>
<body>
</body>
</html>
2. Inside the body tag, create a div element with a class of "container".
<div class="container">
<a href="#" class="icon facebook">
<div class="title">Facebook</div>
<i class="fab fa-facebook"></i>
</a>
<a href="#" class="icon twitter">
<div class="title">Twitter</div>
<i class="fab fa-twitter"></i>
</a>
<a href="#" class="icon instagram">
<div class="title">Instagram</div>
<i class="fab fa-instagram"></i>
</a>
<a href="#" class="icon whatsapp">
<div class="title">WhatsApp</div>
<i class="fab fa-whatsapp"></i>
</a>
<a href="#" class="icon youtube">
<div class="title">YouTube</div>
<i class="fab fa-youtube"></i>
</a>
</div>
3. Create a CSS file and style your Social Media Icon to make it look nice. You can use the following code as a starting point:
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
background: #d3eaff;
font-family: sans-serif;
}
.container .icon {
position: relative;
background: #fff;
color: #222;
border-radius: 5px;
margin: 8px;
width: 48px;
height: 48px;
cursor: pointer;
font-size: 22px;
display: inline-flex;
align-items: center;
justify-content: center;
box-shadow: 0 10px 10px #0002;
transition: all 0.2s ease-in-out;
text-decoration: none;
user-select: none;
}
.container .title {
position: absolute;
top: 0;
line-height: 1.5;
font-size: 13px;
background: #fff;
color: #fff;
padding: 5px 8px;
border-radius: 5px;
box-shadow: 0 10px 10px #0002;
opacity: 0;
pointer-events: none;
transition: all 0.2s ease-in-out;
}
.container .title::before {
position: absolute;
content: "";
height: 8px;
width: 8px;
background: #fff;
bottom: -3px;
left: 50%;
transform: translate(-50%) rotate(45deg);
transition: all 0.2s ease-in-out;
}
.container .icon:hover .title {
top: -45px;
opacity: 1;
visibility: visible;
pointer-events: auto;
}
.container .icon:hover span,
.container .icon:hover .title {
text-shadow: 0 -1px 0 #0002;
}
.container .facebook:hover,
.container .facebook:hover .title,
.container .facebook:hover .title::before {
background: #3b5999;
color: #fff;
}
.container .twitter:hover,
.container .twitter:hover .title,
.container .twitter:hover .title::before {
background: #2b91ff;
color: #fff;
}
.container .instagram:hover,
.container .instagram:hover .title,
.container .instagram:hover .title::before {
background: #f9006c;
color: #fff;
}
.container .whatsapp:hover,
.container .whatsapp:hover .title,
.container .whatsapp:hover .title::before {
background: #008902;
color: #fff;
}
.container .youtube:hover,
.container .youtube:hover .title,
.container .youtube:hover .title::before {
background: #bc0000;
color: #fff;
}
4. Save the file and open it in a web browser to see your Social Media Icon.
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css">
<div class="container">
<a href="#" class="icon facebook">
<div class="title">Facebook</div>
<i class="fab fa-facebook"></i>
</a>
<a href="#" class="icon twitter">
<div class="title">Twitter</div>
<i class="fab fa-twitter"></i>
</a>
<a href="#" class="icon instagram">
<div class="title">Instagram</div>
<i class="fab fa-instagram"></i>
</a>
<a href="#" class="icon whatsapp">
<div class="title">WhatsApp</div>
<i class="fab fa-whatsapp"></i>
</a>
<a href="#" class="icon youtube">
<div class="title">YouTube</div>
<i class="fab fa-youtube"></i>
</a>
</div>
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
background: #d3eaff;
font-family: sans-serif;
}
.container .icon {
position: relative;
background: #fff;
color: #222;
border-radius: 5px;
margin: 8px;
width: 48px;
height: 48px;
cursor: pointer;
font-size: 22px;
display: inline-flex;
align-items: center;
justify-content: center;
box-shadow: 0 10px 10px #0002;
transition: all 0.2s ease-in-out;
text-decoration: none;
user-select: none;
}
.container .title {
position: absolute;
top: 0;
line-height: 1.5;
font-size: 13px;
background: #fff;
color: #fff;
padding: 5px 8px;
border-radius: 5px;
box-shadow: 0 10px 10px #0002;
opacity: 0;
pointer-events: none;
transition: all 0.2s ease-in-out;
}
.container .title::before {
position: absolute;
content: "";
height: 8px;
width: 8px;
background: #fff;
bottom: -3px;
left: 50%;
transform: translate(-50%) rotate(45deg);
transition: all 0.2s ease-in-out;
}
.container .icon:hover .title {
top: -45px;
opacity: 1;
visibility: visible;
pointer-events: auto;
}
.container .icon:hover span,
.container .icon:hover .title {
text-shadow: 0 -1px 0 #0002;
}
.container .facebook:hover,
.container .facebook:hover .title,
.container .facebook:hover .title::before {
background: #3b5999;
color: #fff;
}
.container .twitter:hover,
.container .twitter:hover .title,
.container .twitter:hover .title::before {
background: #2b91ff;
color: #fff;
}
.container .instagram:hover,
.container .instagram:hover .title,
.container .instagram:hover .title::before {
background: #f9006c;
color: #fff;
}
.container .whatsapp:hover,
.container .whatsapp:hover .title,
.container .whatsapp:hover .title::before {
background: #008902;
color: #fff;
}
.container .youtube:hover,
.container .youtube:hover .title,
.container .youtube:hover .title::before {
background: #bc0000;
color: #fff;
}