Template inheritance is a powerful feature provided by the Jinja2 templating engine, which is commonly used with Flask. It allows you to define a base template with common elements and then inherit from it to create child templates that can override or extend specific sections. This helps in reducing duplication and organizing your templates more effectively.
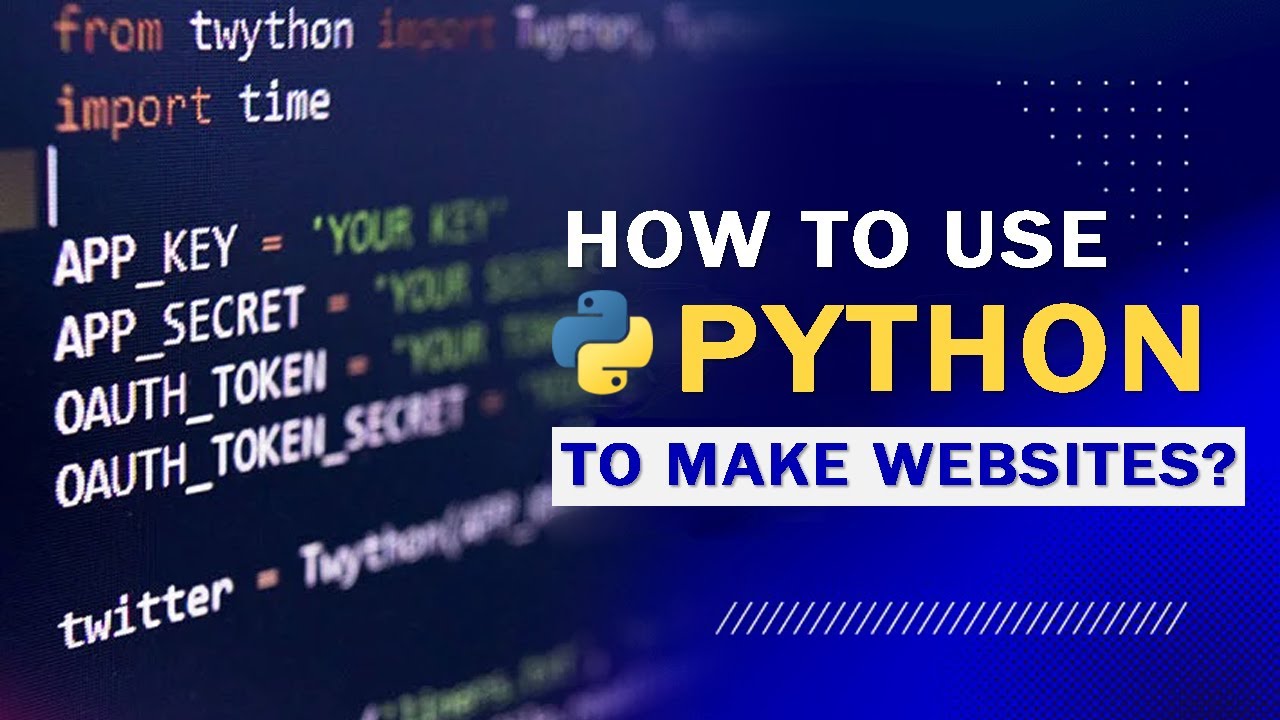
Here's an example to illustrate template inheritance in Jinja2:
Step 1: Create a Base Template
Create a new HTML file called base.html
, which will serve as the base template. It defines the common structure and layout for your website. For example:
<!DOCTYPE html>
<html>
<head>
{% block head %}{% endblock %}
</head>
<body>
<header>
<h1>My Website</h1>
<nav>
<a href="/">Home</a>
<a href="/about">About</a>
</nav>
</header>
<main>
{% block content %}
{% endblock %}
</main>
<footer>
© 2023 My Website
</footer>
</body>
</html>
In this base template, we define blocks using {% block block_name %}{% endblock %}
tags. These blocks act as placeholders that child templates can override or extend.
Step 2: Create a Child Template
Create a new HTML file called home.html
, which will inherit from the base template. It will override the head
and content
blocks. For example:
{% extends 'base.html' %}
{% block head %}<title>My Website</title>{% endblock %}
{% block content %}
<h2>Welcome to my website</h2>
<p>This is the homepage.</p>
{% endblock %}
Step 3: Render the Child Template
In this child template, we use the {% extends 'base.html' %}
tag to indicate that it inherits from the base.html
template. Then, we override the head
and content
blocks with specific content for the home page.
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
Now, when you access the home page of your Flask app, the home.html
template will be rendered and inserted into the corresponding blocks defined in the base.html
template. This way, you have a consistent layout across different pages, and you can easily modify specific sections by overriding the relevant blocks in child templates.
You can create more child templates that inherit from the base.html
template and override or extend different blocks as needed, allowing you to build a complex website with reusable templates.