To fetch posts from a database using Flask SQLAlchemy, you can utilize the query capabilities provided by SQLAlchemy. Here's an example of how you can implement the fetching of posts from a database in a Flask application:
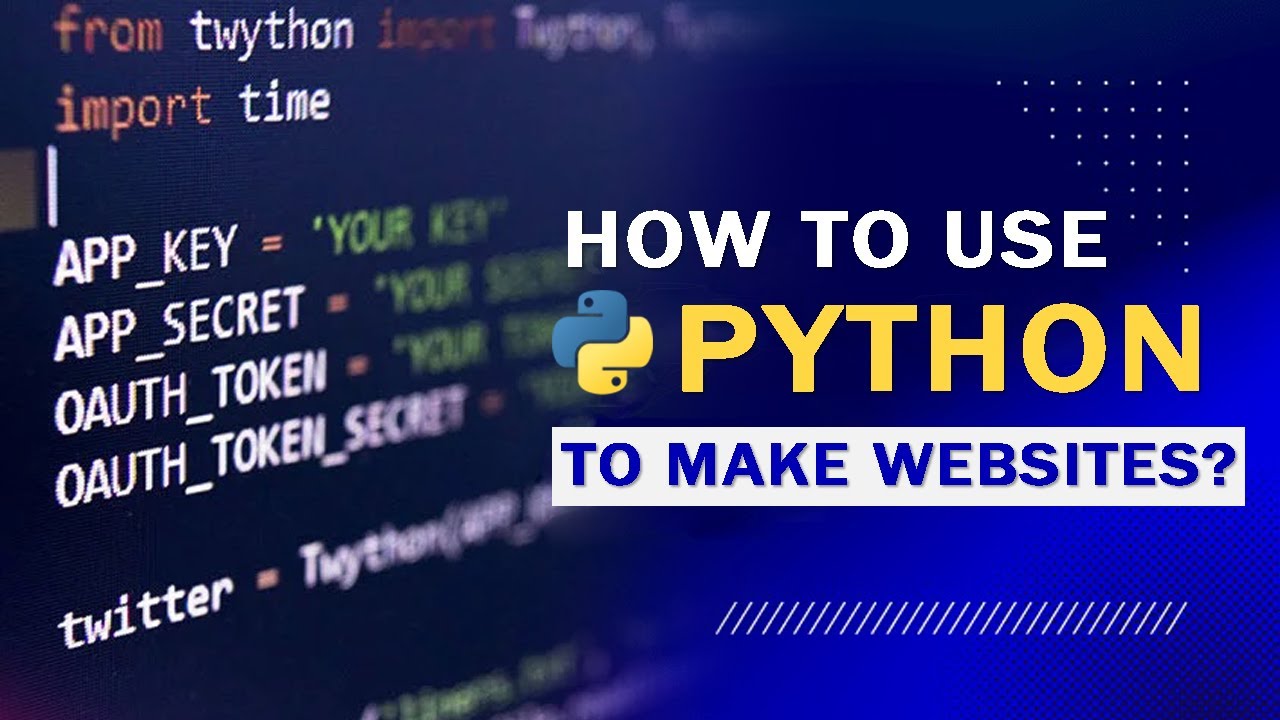
Step 1: Set up Flask and SQLAlchemy
Make sure you have Flask and SQLAlchemy installed. If not, you can install them using the following commands:
pip install Flask-SQLAlchemy
Step 2: Configure Flask and SQLAlchemy
Create a Flask application and configure the SQLAlchemy database connection. For example:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config["SQLALCHEMY_DATABASE_URI"] = "mysql://root:@localhost/pywebsite" # Replace with your database URI
db = SQLAlchemy(app)
Step 3: Define the Post model
Create a model for the posts. Here's an example of a simple Posts
model:
class Posts(db.Model):
sno = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100), nullable=False)
slug = db.Column(db.String(100), nullable=False)
content = db.Column(db.String(), nullable=False)
date = db.Column(db.String(20), nullable=True)
image = db.Column( nullable=True)
Step 4: Fetch posts from the database
Now, you can fetch posts from the database using SQLAlchemy's query capabilities. Here's an example route that retrieves all posts:
import re
def remove_tag(tag):
a = re.compile('<.*?>')
b = re.sub(a, '',tag)
return b
@app.route("/")
def home():
posts = Posts.query.all()
for n in posts:
n.content = remove_tags(n.content)
title = "Welcome to FlaskWeb"
return render_template("home.html", title = title, posts = posts)
In the get_posts()
function, all posts are fetched from the database using Post.query.all()
. The fetched posts are then transformed into a list of dictionaries, and the response is returned as JSON using Flask's jsonify
function.
Step 5: Create a Jinja2 template
Create a Jinja2 template file named template.html
in your templates directory. In this template, you can use a loop to iterate over the posts and display their content. For example:
<!DOCTYPE html>
<html>
<head>
<title>{{title}}</title>
</head>
<body>
<h1>Posts</h1>
<group class="group post-container">
{% for post in posts %}
<div class="index-post">
<a class="image" href="/{{post.slug}}">
<img alt="{{post.title}}" class="img" height="155"
loading="eager|lazy"
src="{{post.image}}"
title="{{post.title}}" width="277">
</a>
<div class="meta">
<h2 class="post-title" >
<a href="/{{post.slug}}"
id="{{post.sno}}"
title="{{post.title}}">{{post.title}}</a>
</h2>
<div class="post-meta msize">
<span class="post-date" datetime="{{post.title}}">{{post.date}}</span>
</div>
<p class="post-snippet">{{post.content[0:150] | safe}}...</p>
</div>
</div>
{% endfor %}
</group>
</body>
In this template, the {% for post in posts %}
loop iterates over the posts
variable, which is passed from the Flask route. Inside the loop, you can access the attributes of each post
object, such as post.title
, post.slug
, post.content
, post.date
and post.image
, to display the post information.
Step 6: Run the Flask application
Save the file and run the Flask application using the following command:
python app.py
Now, you can send a GET request to http://localhost:5000
to retrieve all the posts from the database.
Remember to handle error cases, implement pagination or filtering if necessary, and customize the fetching logic based on your specific requirements.